Simple webhook to execute local scripts
Simple Golang app that executes a script from a webhook
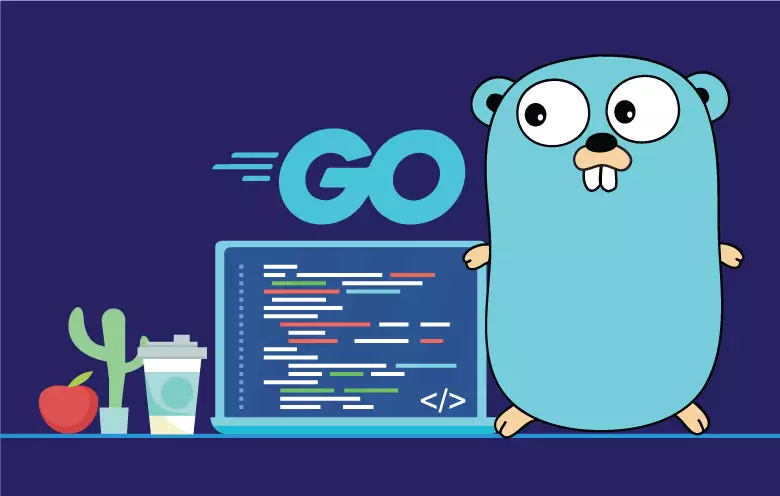
Many web apps run on separate devices, we can automate specific tasks using web hooks. Webhooks are automated messages sent from web applications when specific events occur. They allow different systems or services to communicate with each other in real-time. Here's a simple breakdown:
- Trigger Event: An event happens in one application, such as a new user signing up.
- HTTP POST: This event triggers a predefined HTTP POST request to a specified URL (the webhook URL).
- Receiver: The server at the webhook URL receives the POST request and processes the payload (data) sent with it.
- Action: Based on the received data, the receiving server can take actions like updating a database, sending notifications, or triggering further processes.
While Distros such as Debian use the webhooks project "webhook" it's often simpler to run a basic GO app that takes the post request and executes the needed script.
package main
import (
"fmt"
"os/exec"
"net/http"
)
func main() {
http.HandleFunc("/webhook", func(w http.ResponseWriter, r *http.Request) {
if r.Method!= http.MethodPost {
http.Error(w, "Invalid request method", http.StatusMethodNotAllowed)
return
}
// Define the path to the script
scriptPath := "/etc/nginx/scripts/clear.sh"
// Execute the script
cmd := exec.Command("/bin/sh", scriptPath)
output, err := cmd.CombinedOutput()
if err!= nil {
fmt.Printf("Error executing script: %v\n", err)
http.Error(w, "Failed to execute script", http.StatusInternalServerError)
return
}
// Print the output of the script
fmt.Printf("Script output:\n%s\n", string(output))
// Respond to the webhook call
w.WriteHeader(http.StatusOK)
w.Write([]byte("OK"))
})
fmt.Println("Server starting on :8080...")
if err := http.ListenAndServe(":8080", nil); err!= nil {
fmt.Printf("Failed to start server: %v\n", err)
}
}